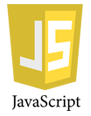
JavaScript VoIP developers 101
Part 2: Voice call between a webbrowser and a VoIP phone
Study the following article to get more information about doing basic tasks in the Ozeki Phone System by using your JavaScript application. This guide presents how to call a VoIP phone from a webbrowser.
- Download example project: ozeki-voicecall.zip (0.75 KB)
- Reference manual: http://www.ozekiphone.com/examples/jsdoc/index.html
1. What you need
- A text editor (for example Notepad++)
- Ozeki Phone System installed on your PC (Download now)
- A VoIP phone SIP extension installed on Ozeki Phone System:
2. Get started
- Create a Webphone outside line in Ozeki Phone System
- Get IP address and port of your PBX (e.g. http://ozekixepbx.ip:7777/)
- Create a html document
- Add reference to WebphoneAPI
(e.g. <script type="text/javascript" src="http://ozekixepbx.ip7777/WebphoneAPI"></script>)
User manual
The Ozeki Phone System makes it possible to connect the customers and the appropriate extensions together through a fully customisable WebPhone. It can be done by using your PBX and the configurable dial plan rules. For this purpose you only need to install a Webphone outside line in the Ozeki Phone Sytem XE and add the required routing rule. If the default dial plan rule has been added, there is no need to specify the called number before making a call. In addition, it is also possible to dial a previously installed extension or an outside line number directly by entering its telephone number. How to setup a Webphone outside line
For using WebPhoneAPI a webserver is essentially needed on which your written code will be executable. Any webserver can be used, just select one and download it. In this example Wampserver and Apache was used. After installing WampServer, you need to modify the 'Listen 80' value in the httpd.conf file belonging to Apache in order to avoid port collision. Rewrite it to 'Listen 8080' (or to any other number that is not used by other applications). Do the modification then restart the service. After that open the folder where you have installed Wamp then select the 'www' folder. Here create a 'voicecall(browser-VoIP).html' file and put the following content in it:
<!DOCTYPE html> <html> <head> <script type="text/javascript"> </script> </head> <body> </body> </html> |
The JavaScript code which you will write needs to be placed between <script> </script> tags.
Implementation
Before developing JavaScript, reference the WebphoneAPI JavaScript file into your source. Place the following row directly after the opening <head> tag:
<script type="text/javascript" |
In this script the 'ozekixepbx.ip' and the 'PBX_ServerPort' needs to be the server address and port number of Ozeki Phone System configured previously. The default port number is 7777.
3. Create a JavaScript code calling up a VoIP phone from a webbrowser
- Connect to the webphone outside line
- Create a call to the VoIP phone
This code will work after you have connected a VoIP phone to your Ozeki Phone System. This can be a desktop VoIP phone or a softphone installed on a PC.
Read VoIP phone installation guides here:
- How to connect a desktop VoIP phone to Ozeki Phone System
- How to connect a softphone to Ozeki Phone System
After this process the VoIP phone will have a SIP extension number, use this phone number in the JavaScript to call the VoIP phone from the webbrowser.
At the start of the code we need to declare a call class object which we will call MyCall. This is how we declared it: var MyCall = null; This object will represent the call.
Connect to the webphone outside line
After declaring the MyCall object we wait a short period of time for example 0.2 seconds till the page is loaded then we call the begin() function. This function registers to the onConnectionStateChanged event and tries to connect to the webphone outside line.
The onConnectionStateChanged event occurs when the state of the connection changes.
It can have 4 states:
"ACCESS_GRANTED", "ACCESS_DENIED", "CONNECTION_FAILED", "CONNECTION_CLOSED".
It is vital to notice when the webclient has connected to the webphone outside line.
You can connect to the webphone outside line by using the connect method . As a parameter of this method you need to provide the IP address of the
Ozeki Phone System you use and the name of the webphone outside line (Code Example 3).
setTimeout('begin()', 200); //wait 0.2 seconds function begin() { OzWebClient.onConnectionStateChanged(connectionStateChanged); OzWebClient.connect("192.168.115.131", "Webphone1"); } |
Create a call to the VoIP phone
When an onConnectionStateChanged event occurs the parameter of the event is called, which is in our case the connectionStateChanged function. This function gets the current connectionstate in its state parameter. If this state parameter equals the "ACCESS_GRANTED" string then the webclient has been successfully connected. Now its time to create the call. Use the phone number of the VoIP phone as a parameter in the createCall method. This method makes a call object called MyCall. Run the start method on this object to call the VoIP phone. Then register on the onCallStateChanged event with the callStateChanged function.
If you check the callStateChanged function, you can see that if the call is not in "RINGING", "IN_CALL" or "HOLD" state then the call will be hung up and the webclient will be disconnected. (Code Example 4).
function connectionStateChanged(info) { console.log(info.State); if (info.State == ConnectionState.ACCESS_GRANTED) { MyCall = OzWebClient.createCall("1000"); //target VoIP phone number MyCall.start(); MyCall.onCallStateChanged(callStateChanged); console.log("calling " + MyCall.getOtherParty() + "..."); } } function callStateChanged(state) { console.log(state); if (state != "RINGING" && state != "IN_CALL" && state != "HOLD") { setTimeout(hangUpCall, 1000); } } function hangUpCall() { if (MyCall) { MyCall.hangUp(); console.log("The call is hung up."); MyCall = null; } OzWebClient.disconnect(); } |
4. Create a more advanced project
The Ozeki Phone System offers a lot more options for JavaScript developers. You can interact
with existing calls, control and configure the PBX, you can introduce new communication
techniques and media formats.
For a complete list of JavaScript commands, check out:
JavaScript API reference book
If you have any questions or need assistance, please contact us at info@ozekiphone.com
More information